SHT31-D Temperature and Humidity Sensor
Here is a uLisp function to read the temperature and relative humidity from an I2C SHT31-D sensor which provides an accuracy of ±2% RH and ± 0.3°C:
(defun sht31-temp-humid ()
(let ((rdword
(lambda (s)
(logior (ash (read-byte s) 8) (read-byte s)))))
; High repeatability mode
(with-i2c (s #x44)
(write-byte #x24 s)
(write-byte #x00 s)
(delay 20)
(restart-i2c s 5)
(let* ((temp (rdword s))
(checksum (read-byte s))
(humid (rdword s)))
(list
(- (/ (* temp 175) 65535) 45)
(/ (* humid 100) 65535))))))
It returns the values as a list of two floating-point numbers. For example:
> (sht31-temp-humid)
(20.132 38.1659)
The last two lines of the routine could probably be rewritten to return 16-bit fixed-point values, to make it compatible with 16-bit uLisp platforms.
The sensor also incorporates a heater, to combat condensation. Here’s a routine to enable or disable the heater:
(defun sht31-heater (on)
(with-i2c (s #x44)
(write-byte #x30 s)
(write-byte (if on #x6D #x66) s)))
An SHT31-D Temperature and Humidity Sensor breakout board is available from Adafruit:
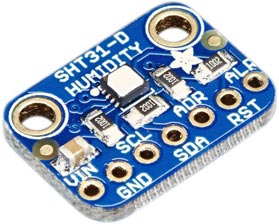
Adafruit Sensirion SHT31-D Temperature & Humidity Sensor Breakout